Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
プレイヤーの入力を聞く¶
Building upon the previous lesson, 初めてのスクリプト作成, let's look
at another important feature of any game: giving control to the player.
To add this, we need to modify our sprite_2d.gd
code.
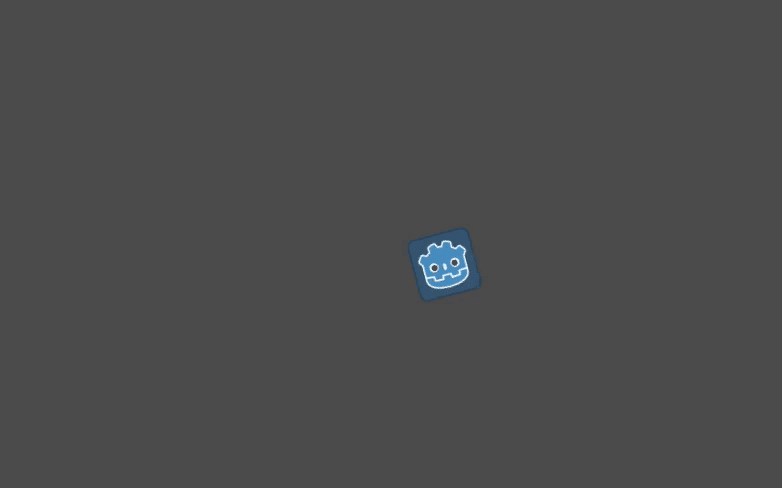
Godotでは、プレイヤーの入力を処理するために、主に2つのツールが用意されています。
組み込みの入力コールバック。主に
_unhandled_input()
です。これは、_process()
のように、プレーヤーがキーを押すたびに Godot が呼び出す組み込みの仮想関数です。例えば Space を押してジャンプするような、毎フレームで発生しないイベントに反応するために使用するツールです。入力コールバックについて詳しくは、 Using InputEvent を参照してください。Input
シングルトン。シングルトンは、グローバルにアクセス可能なオブジェクトです。Godotはスクリプトでいくつかへのアクセスを提供しています。これは、毎フレーム入力があるかどうかを確認するのに適したツールです。
ここでは、Input
シングルトンを使用します。これは、プレイヤーがフレームごとに回転または移動したいかを知る必要があるからです。
回転させるために、新しい変数を使用する必要があります。これをdirection
とします。_process()
関数の rotation += angular_speed * delta
行を以下のコードに置き換えてください。
var direction = 0
if Input.is_action_pressed("ui_left"):
direction = -1
if Input.is_action_pressed("ui_right"):
direction = 1
rotation += angular_speed * direction * delta
var direction = 0;
if (Input.IsActionPressed("ui_left"))
{
direction = -1;
}
if (Input.IsActionPressed("ui_right"))
{
direction = 1;
}
Rotation += _angularSpeed * direction * (float)delta;
ローカル変数direction
は、プレイヤーが曲がりたい方向を表す乗数です。値が0
なら、プレーヤーが左または右矢印キーを押していないことを意味します。値1
なら、プレイヤーが右に曲がりたい、-1
なら左に曲がりたいことを意味します。
これらの値を生成するために、条件とInput
の使用を導入します。条件は、GDScriptのif
キーワードで始まり、コロンで終わります。条件は、キーワードと行末の間の式です。
このフレームでキーが押されたかどうかを確認するために、Input.is_action_pressed()
を呼び出します。このメソッドは入力アクションを表す文字列を受け取り、アクションが押された場合はtrue
を、それ以外の場合はfalse
を返すようになっています。
上記で使用した2つのアクション、"ui_left" と "ui_right" は、すべてのGodotプロジェクトであらかじめ定義されています。それぞれ、プレイヤーがキーボードの左矢印と右矢印、またはゲームパッドのDパッドの左と右を押したときに起動します。
注釈
プロジェクト("Project") -> プロジェクト設定("Project Settings") から インプットマップ("Input Map") タブをクリックすると、プロジェクト内の入力アクションを確認・編集することができます。
最後に、direction
をノードのdirection
の更新時の乗数として使用します。rotation += angular_speed * direction * delta
とします。
このコードでシーンを実行すると、 Left と Right を押したときにアイコンが回転します。
「上」ボタンを押すと動く¶
キーを押した時だけ動くようにするには、速度を計算するコードを修正する必要があります。var velocity
で始まる行を、以下のコードに置き換えます。
var velocity = Vector2.ZERO
if Input.is_action_pressed("ui_up"):
velocity = Vector2.UP.rotated(rotation) * speed
var velocity = Vector2.Zero;
if (Input.IsActionPressed("ui_up"))
{
velocity = Vector2.Up.Rotated(Rotation) * _speed;
}
velocity
をVector2.ZERO
という値で初期化します。これはVector
型の組み込み定数の一つで、長さ0の2次元ベクトルを表します。
プレイヤーが"ui_up"アクションを押すと、velocityの値が更新され、スプライトが前に移動するようになります。
完全なスクリプト¶
Here is the complete sprite_2d.gd
file for reference.
extends Sprite2D
var speed = 400
var angular_speed = PI
func _process(delta):
var direction = 0
if Input.is_action_pressed("ui_left"):
direction = -1
if Input.is_action_pressed("ui_right"):
direction = 1
rotation += angular_speed * direction * delta
var velocity = Vector2.ZERO
if Input.is_action_pressed("ui_up"):
velocity = Vector2.UP.rotated(rotation) * speed
position += velocity * delta
using Godot;
public partial class Sprite : Sprite2D
{
private float _speed = 400;
private float _angularSpeed = Mathf.Pi;
public override void _Process(double delta)
{
var direction = 0;
if (Input.IsActionPressed("ui_left"))
{
direction = -1;
}
if (Input.IsActionPressed("ui_right"))
{
direction = 1;
}
Rotation += _angularSpeed * direction * (float)delta;
var velocity = Vector2.Zero;
if (Input.IsActionPressed("ui_up"))
{
velocity = Vector2.Up.Rotated(Rotation) * _speed;
}
Position += velocity * (float)delta;
}
}
シーンを実行すると、左右の矢印キーで回転し、 Up を押して前進することができるはずです。
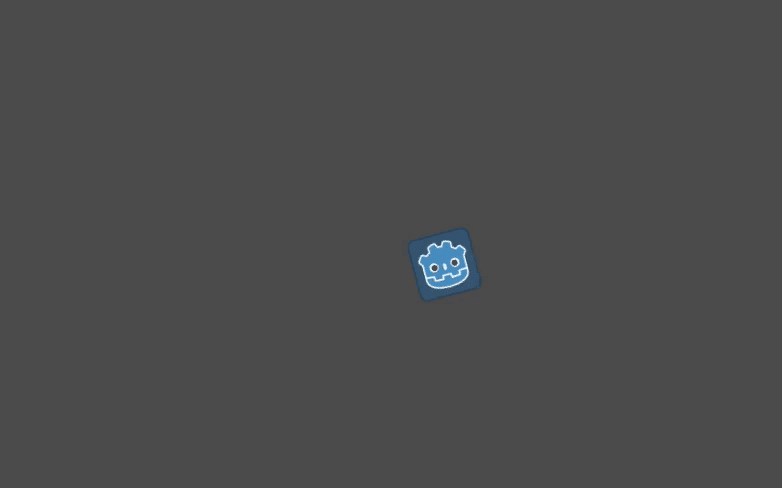
概要¶
In summary, every script in Godot represents a class and extends one of the
engine's built-in classes. The node types your classes inherit from give you
access to properties, such as rotation
and position
in our sprite's case.
You also inherit many functions, which we didn't get to use in this example.
GDScript では、ファイルの先頭に置いた変数は、クラスのプロパティで、メンバー変数とも呼ばれます。また、変数以外にも関数を定義することができますが、これはほとんどの場合、クラスのメソッドになります。
Godotは、クラスとエンジンを接続するために定義可能ないくつかの仮想関数を提供します。例えば、_process()
はフレームごとにノードに変更を適用し、_unhandled_input()
はユーザからキーやボタンを押すなどの入力イベントを受け取ります。他にもたくさんの仮装関数があります。
Input
シングルトンにより、コードの任意の場所でプレーヤーの入力に反応することができます。特に、_process()
のループで使用することになります。
In the next lesson, シグナルの使用, we'll build upon the relationship between scripts and nodes by having our nodes trigger code in scripts.