Creating Android plugins¶
はじめに¶
Androidプラグインは、Androidプラットフォームとエコシステムによって提供される機能を活用して、Godotエンジンの機能を拡張する強力なツールです。
モバイルゲームの収益化は、ゲームエンジンのコア機能セットに属さない機能を必要とするため、そのような例の1つです:
分析
アプリ内購入
証明書の検証
トラッキングのインストール
広告
動画広告
クロスプロモーション
ゲーム内のソフト通貨とハード通貨
プロモーションコード
A/B テスト
ログイン
クラウド保存
ランキングとスコア
ユーザーサポートとフィードバック
Facebook、Twitterなどへの投稿。
プッシュ通知
Androidプラグイン¶
While introduced in Godot 3.2, the Android plugin system got a significant architecture update starting with Godot 3.2.2.
The new plugin system is backward-incompatible with the previous one, but both systems are kept functional in future releases of the 3.2.x branch.
Since we previously did not version the Android plugin systems, the new one is now labelled v1
and is the starting point for the modern Godot Android ecosystem.
Note: In Godot 4.0, the previous system will be fully deprecated and removed.
前提条件として、Android用に カスタムビルド環境 をセットアップする方法を理解してください。
基本的に、Godot Androidプラグインは `Androidアーカイブライブラリ <https://developer.android.com/studio/projects/android-library#aar-contents>`_(aar アーカイブファイル)であり、次の注意事項があります:
The library must have a dependency on the Godot engine library (
godot-lib.<version>.<status>.aar
). A stable version is made available for each Godot release on the Godot download page.ライブラリには、マニフェストファイルに特別に構成された
<meta-data>
タグを含める必要があります。
Building an Android plugin¶
前提条件: Androidプラグインの作成に使用するIDEとして、Android Studio を強く推奨します。以下の手順は、Android Studioを使用していることを前提としています。
これらの手順 に従ってプラグイン用のAndroidライブラリモジュールを作成します。
プラグインモジュールへの依存関係としてGodotエンジンライブラリを追加します:
Download the Godot engine library (
godot-lib.<version>.<status>.aar
) from the Godot download page (e.g.:godot-lib.3.4.2.stable.release.aar
).これらの手順 に従って、プラグインの依存関係としてGodotエンジンライブラリを追加します。
プラグインモジュールの
build.gradle
ファイルで、Godotエンジンライブラリの依存関係行のimplementation
をcompileOnly
に置き換えます。
Create a new class in the plugin module and make sure it extends
org.godotengine.godot.plugin.GodotPlugin
. At runtime, it will be used to instantiate a singleton object that will be used by the Godot engine to load, initialize and run the plugin.プラグイン
AndroidManifest.xml
ファイルを更新します:
プラグイン
AndroidManifest.xml
ファイルを開きます。存在しない場合は、
<application></application>
タグを追加します。
<application>
タグで、次のように<meta-data>
タグの設定を追加します:<meta-data android:name="org.godotengine.plugin.v1.[PluginName]" android:value="[plugin.init.ClassFullName]" />ここで、
PluginName
はプラグインの名前であり、plugin.init.ClassFullName
はプラグイン読み込みクラスのフルネーム(パッケージ+クラス名)です。
プラグインの残りのロジックを追加し、
gradlew build
コマンドを実行してプラグインのaar
ファイルを生成します。ビルドはおそらくdebug
とrelease
aar``ファイルの両方を生成します。必要に応じて、ユーザーに提供するバージョンを1つだけ選択します(通常は ``release
バージョン)。It's recommended that the
aar
filename matches the following pattern:[PluginName]*.aar
wherePluginName
is the name of the plugin in PascalCase (e.g.:GodotPayment.release.aar
).Create a Godot Android Plugin configuration file to help the system detect and load your plugin:
The configuration file extension must be
gdap
(e.g.:MyPlugin.gdap
).The configuration file format is as follow:
[config] name="MyPlugin" binary_type="local" binary="MyPlugin.aar" [dependencies] local=["local_dep1.aar", "local_dep2.aar"] remote=["example.plugin.android:remote-dep1:0.0.1", "example.plugin.android:remote-dep2:0.0.1"] custom_maven_repos=["http://repo.mycompany.com/maven2"]The
config
section and fields are required and defined as follow:
name: (プラグインの名前)。
binary_type: can be either
local
orremote
. The type affects the binary field.binary:
If binary_type is
local
, then this should be the filepath of the pluginaar
file.
The filepath can be relative (e.g.:
MyPlugin.aar
) in which case it's relative to theres://android/plugins
directory.The filepath can be absolute:
res://some_path/MyPlugin.aar
.If binary_type is
remote
, then this should be a declaration for a remote gradle binary (e.g.:org.godot.example:my-plugin:0.0.0
).The
dependencies
section and fields are optional and defined as follow:
local: contains a list of filepaths to the local
.aar
binary files the plugin depends on. Similarly to thebinary
field (when thebinary_type
islocal
), the local binaries' filepaths can be relative or absolute.remote: contains a list of remote binary gradle dependencies for the plugin.
custom_maven_repos: contains a list of URLs specifying the custom maven repositories required for the plugin's dependencies.
Loading and using an Android plugin¶
Move the plugin configuration file (e.g.: MyPlugin.gdap
) and, if any, its local binary (e.g.: MyPlugin.aar
) and dependencies to the Godot project's res://android/plugins
directory.
The Godot editor will automatically parse all .gdap
files in the res://android/plugins
directory and show a list of detected and toggleable plugins in the Android export presets window under the Plugins section.
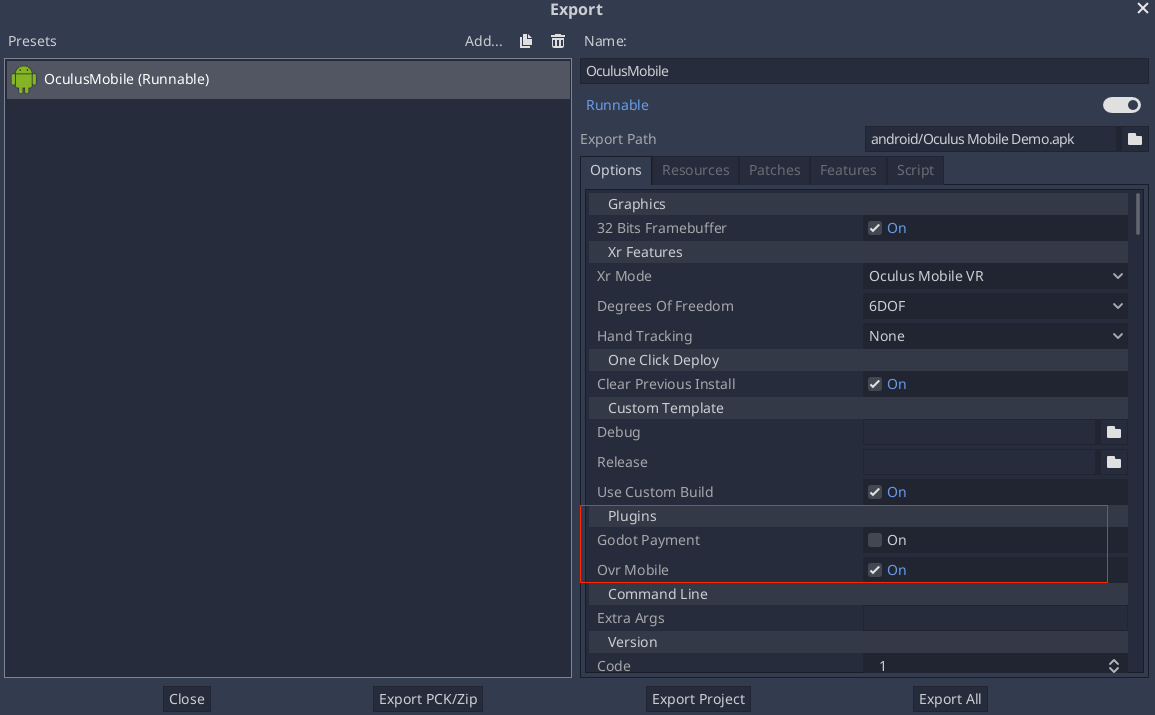
From your script:
if Engine.has_singleton("MyPlugin"):
var singleton = Engine.get_singleton("MyPlugin")
print(singleton.myPluginFunction("World"))
GDNativeリソースのバンドル¶
An Android plugin can define and provide C/C++ GDNative resources, either to provide and/or access functionality from the game logic.
The GDNative resources can be bundled within the plugin aar
file which simplifies the distribution and deployment process:
定義されたGDNativeライブラリの共有ライブラリ(
.so
)は、aar
ビルドシステムによって自動的にバンドルされます。Godotの
*.gdnlib
および*.gdns
リソースファイルは、プラグインのassets
ディレクトリで手動で定義する必要があります。assets
ディレクトリに対するこれらのリソースの推奨パスは、godot/plugin/v1/[PluginName]/
でなければなりません。
GDNativeライブラリの場合、プラグインシングルトンオブジェクトは org.godotengine.godot.plugin.GodotPlugin::getPluginGDNativeLibrariesPaths()
メソッドをオーバーライドし、バンドルされたGDNativeライブラリ設定ファイル(*.gdnlib
へのパスを返す必要があります)。パスは assets
ディレクトリからの相対パスでなければなりません。実行時に、プラグインはこれらのパスをGodotコアに提供します。Godotコアはそれらを使用して、バンドルされたGDNativeライブラリをロードおよび初期化します。
リファレンス実装¶
トラブルシューティング¶
ロード時にGodotがクラッシュする¶
adb logcat
で問題がないかチェックしてください:
Check that the methods exposed by the plugin used the following Java types:
void
,boolean
,int
,float
,java.lang.String
,org.godotengine.godot.Dictionary
,int[]
,byte[]
,float[]
,java.lang.String[]
.More complex datatypes are not supported for now.